Draft for Information Only
Content
Python Dictionary Characteristics of Dictionary Ordered Changeable No Duplicates Operations of Dictionary Item Manipulation Single Item Manipulation Multiple Items Manipulation Dictionary Manipulation Dictionary Generation Other Iterator Generation Dictionary view objects Source and Reference
Python Dictionary
A dictionary is a collection of key:value pairs enclosed by curly brackets.
Characteristics of Dictionary
Items of a dictionary are ordered, changeable and no duplicates.
Ordered
Originally, dictionaries are unordered sets in Python. But now, dictionaries are a collection of ordered items.
Changeable
The value of a specific item in a dictionary can be changed by referring to its key.
No Duplicates
All keys in a dictionary must be unique and no duplicate is allowed.
Operations of Dictionary
d={key:value}
Item Manipulation
Single Item Manipulation
d[key]Return the item value of item in d with item key key or raises a KeyError if key is not in the map.
d[key] = valueSet the item value of item in d with item key key.
del d[key]Remove the item from d with item key key or raises a KeyError if key is not in the map.
key in dReturn True if d has a item key key, else False.
key not in dReturn False if d has a item key key, else True.Equivalent to not key in d.
d.get( key[, default])Return the item value for item key key if item key key is in the dictionary, else default. The value of default is equal to None, if default is not given.
d.setdefault( key[, default])Return the item value for item key key if item key key is in the dictionary, else return default and insert item with item key key and item value to d. The value of default is equal to None, if default is not given.
d.pop(key[, default])Return the item value and remove the item with item key key in the dictionary if the item key key is in the dictionary, else return default. Or raise a KeyError, if default is not given and key is not in the dictionary,
d.popitem()Return a (key, value) pair and remove the item from the dictionary. Popitem are returned in LIFO order. Raise a KeyError if the dictionary is empty.
Changed in version 3.7: LIFO order is now guaranteed. In prior versions, popitem() would return an arbitrary key/value pair. popitem() is useful to destructively iterate over a dictionary, as often used in set algorithms.
Multiple Items Manipulation
d.clear()Remove all items from the dictionary.
len(d)Return the number of items in the dictionary d.
d.update( [other])Update the dictionary with the key/value pairs from other, overwriting existing keys. Return None. update() accepts either another dictionary object or an iterable of key/value pairs (as tuples or other iterables of length two). If keyword arguments are specified, the dictionary is then updated with those key/value pairs: d.update(red=1, blue=2).
d |= otherUpdate the dictionary d with keys and values from other, which may be either a mapping or an iterable of key/value pairs. The values of other take priority when d and other share keys.New in version 3.9
Dictionary Manipulation
Dictionary Generation
d.copy()Return a shallow copy of the dictionary.
classmethodd.fromkeys( iterable[, value]) Create a new dictionary with keys from iterable and values set to value. fromkeys() is a class method that returns a new dictionary. value defaults to None. All of the values refer to just a single instance, so it generally doesn’t make sense for value to be a mutable object such as an empty list. To get distinct values, use a dict comprehension instead.
d | otherCreate a new dictionary with the merged keys and values of d and other, which must both be dictionaries. The values of other take priority when d and other share keys.New in version 3.9
Other Iterator Generation
list(d)Return a list of all the keys used in the dictionary d.
iter(d)Return an iterator over the keys of the dictionary. This is a shortcut for iter(d.keys()).
d.items()Return a new view of the dictionary’s items ((key, value) pairs). See the documentation of view objects.
d.keys()Return a new view of the dictionary’s keys. See the documentation of view objects.
reversed(d)Return a reverse iterator over the keys of the dictionary. This is a shortcut for reversed(d.keys()).New in version 3.8
d.values()Return a new view of the dictionary’s values. See the documentation of view objects. An equality comparison between one dict.values() view and another will always return False. This also applies when comparing dict.values() to itself:
>>>
>>> d = {'a': 1}
>>> d.values() == d.values()
False
Dictionary view objects
The objects returned by dict.keys(), dict.values() and dict.items() are view objects. They provide a dynamic view on the dictionary’s entries, which means that when the dictionary changes, the view reflects these changes.
len(dictview)
Return the number of entries in the dictionary.
iter(dictview)
Return an iterator over the keys, values or items (represented as tuples of (key, value)) in the dictionary.
Keys and values are iterated over in insertion order. This allows the creation of (value, key) pairs using zip(): pairs = zip(d.values(), d.keys()). Another way to create the same list is pairs = [(v, k) for (k, v) in d.items()].
Iterating views while adding or deleting entries in the dictionary may raise a RuntimeError or fail to iterate over all entries.
Changed in version 3.7: Dictionary order is guaranteed to be insertion order.
x in dictview
Return True if x is in the underlying dictionary’s keys, values or items (in the latter case, x should be a (key, value) tuple).
reversed(dictview)
Return a reverse iterator over the keys, values or items of the dictionary. The view will be iterated in reverse order of the insertion.
Changed in version 3.8: Dictionary views are now reversible.
Keys views are set-like since their entries are unique and hashable. If all values are hashable, so that (key, value) pairs are unique and hashable, then the items view is also set-like. (Values views are not treated as set-like since the entries are generally not unique.) For set-like views, all of the operations defined for the abstract base class collections.abc.Set are available (for example, ==, <, or ^).
Source and Reference
©sideway
ID: 210500008 Last Updated: 5/8/2021 Revision: 0
|
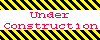 |