enumerate, reversed, sorted, zipfilter, iter, mapabs, divmod, pow, roundbin, chr, hex, oct, ord
Draft for Information Only
Content
Python Built-in Conversion Functions enumerate() Parameters Remarks reversed() Parameters Remarks sorted() Parameters Remarks zip() Parameters Remarks Source and Reference
Python Built-in Conversion Functions
The Python interpreter has some built-in conversion functions.
enumerate()
enumerate(iterable, start=0)
Parameters
type()to return an enumerate object.
iterableto specify the iterable be returned from
start=0to specify the starting number
Remarks
iterable must be a sequence, an interator, or some other object which supports iteration.
- The
__next__() method of the iterator returned by enumerate() returns a tuple containing a count (from start which defaults to 0) and the values obtained from iterating over iterable.
- Equivalent to:
def enumerate(sequence, start=0):
n = start
for elem in sequence:
yield n, elem
n += 1
reversed()
reversed(seq)
Parameters
reversed()to return a reverse iterator.
seqto specify the seq to be returned from
Remarks
- Return a reverse iterator. seq must be an object which has a __reversed__() method or supports the sequence protocol (the __len__() method and the __getitem__() method with integer arguments starting at 0).
sorted()
sorted(iterable, *, key=None, reverse=False)
Parameters
sorted()to return a new sorted list from element of iterable.
iterableto specify the iterable to be returned from
*
key=Noneto specify the comparison key to be used.
reverse=Falseto specify the sorting method.
Remarks
- Return a new sorted list from the items in iterable.
Has two optional arguments which must be specified as keyword arguments.
key specifies a function of one argument that is used to extract a comparison key from each element in iterable (for example, key=str.lower). The default value is None (compare the elements directly).
reverse is a boolean value. If set to True, then the list elements are sorted as if each comparison were reversed.
Use functools.cmp_to_key() to convert an old-style cmp function to a key function.
The built-in sorted() function is guaranteed to be stable. A sort is stable if it guarantees not to change the relative order of elements that compare equal — this is helpful for sorting in multiple passes (for example, sort by department, then by salary grade).
zip()
zip(*iterables)
Parameters
zip()to return an iterator of tuples.
*iterablesto specify the iterables to be returned from.
Remarks
- Make an iterator that aggregates elements from each of the iterables.
Returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument sequences or iterables. The iterator stops when the shortest input iterable is exhausted. With a single iterable argument, it returns an iterator of 1-tuples. With no arguments, it returns an empty iterator. Equivalent to:
def zip(*iterables):
# zip('ABCD', 'xy') --> Ax By
sentinel = object()
iterators = [iter(it) for it in iterables]
while iterators:
result = []
for it in iterators:
elem = next(it, sentinel)
if elem is sentinel:
return
result.append(elem)
yield tuple(result)
The left-to-right evaluation order of the iterables is guaranteed. This makes possible an idiom for clustering a data series into n-length groups using zip(*[iter(s)]*n). This repeats the same iterator n times so that each output tuple has the result of n calls to the iterator. This has the effect of dividing the input into n-length chunks.
zip() should only be used with unequal length inputs when you don’t care about trailing, unmatched values from the longer iterables. If those values are important, use itertools.zip_longest() instead.
zip() in conjunction with the * operator can be used to unzip a list:
Source and Reference
©sideway
ID: 200602302 Last Updated: 6/23/2020 Revision: 0
|
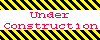 |